How to create an ecommerce single item cart with checkout integrations
Guys today i am going to teach you guys how to build an ecommerce site with checkout integration but only with a single product, you can add more to itSo we are building it using my favorite PHP,Ajax and JQuery.
I have already created a simple shopping cart code in PHP with the product gallery.let's get this over with
What are we building?
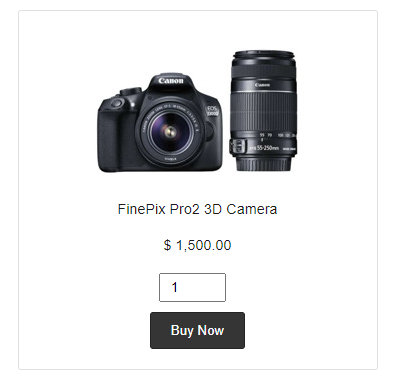
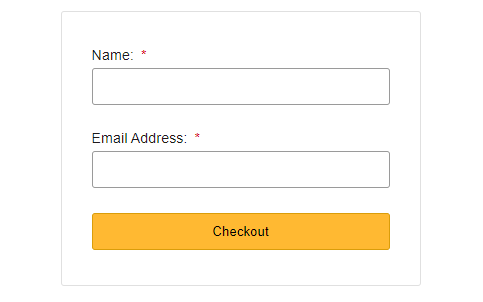
Single product UI with buy now and Checkout controls
This is the code of the landing page created for this example.It includes PHP snippets at the beginning. After that, it has the HTML for displaying only one product tile to users.
This tile has the “Buy now” button. On clicking it will show an HTML form to collect the customer details, name and email address.
By submitting the customer details, it calls the form validation script and the AJAX request to checkout the product.
The initial PHP script links the configuration file and gets the product data from there. The UI embeds the dynamic data into the container using PHP.
The following file structure shows the files created for this eCommerce example.
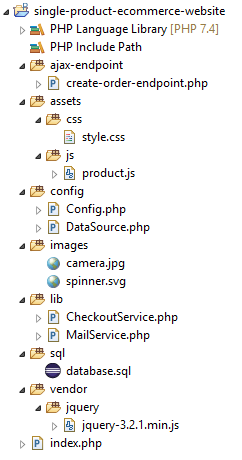
index.php
getProduct(); ?> <HTML> <HEAD>< <TITLE>Single Product ecommerce cart with pur checkout payment</TITLE>
<link href="assets/css/style.css" type="text/css" rel="stylesheet" />
<script src="vendor/jquery/jquery-3.2.1.min.js"></script>
</HEAD>
<BODY>
<div class="txt-heading">Single product eCommerce application</div> <div id="product-grid">
<div class="product-item">
<div>
<img src="<?php echo $configResult["imageUrl"]; ?>">
</div>
<div class="product-tile-footer">
<div class="product-title"><?php echo $configResult["name"]; ?>
</div>
<div class="product-price">
<?php echo "$ ".
number_format($configResult["price"],2);
?>
</div>
<?php if(Config::DISPLAY_QUANTITY_INPUT == true){?>
<div class="quantity"> <input type="text" class="product-quantity" id="productQuantity" name="quantity" value="1" size="2" />
</div><?php }?>
<div> <button name="data" id="btn" onClick="buynow();" class="btnAddAction">Buy Now</button>
</div>
</div>
</div>
</div>
<div id="customer-detail"> <div class="txt-heading">Customers Details
</div>
<div class="product-item">
<form method="post" action="" id="checkout-form">
<div class="row">
<div class="form-label">
Name:<span class="required error" id="first-name-info"></span>
</div>
<input class="input-box" type="text" name="first-name" id="first-name">
</div>
<div class="row">
<div class="form-label">
Email Address: <span class="required error" id="email-info"></span>
</div>
<input class="input-box" type="text" name="email" id="email">
</div>
<div class="row">
<div id="inline-block">
<img id="loader" src="images/spinner.svg" />
<input type="button" class="checkout" name="checkout" id="checkout-btn" value="Checkout" onClick="Checkout();">
</div>
</div>
</form>
</div>
</div>
<div id="mail-status"></div>
< script src="assets/js/shop.js"></script>
</BODY>
</HTML>
jQuery form validation and AJAX checkout
This simple script displays the functions to validate the checkout form and proceed to send the request to the server.The validateUser() function gets the UI input field data and does the validation. It confirms that the fields are not empty and the email is in the right format.
On successful validation, it invokes the Checkout() function which uses jQuery AJAX to send the checkout request to the PHP.
It sends the customer details and the purchased product details as the AJAX parameters.
assets/js/shop.js>
function validateUser() { var valid = true; $("#first-name").removeClass("error-field"); $("#email").removeClass("error-field");
var firstName = $("#first-name").val();
var email = $("#email").val();
var emailRegex = /^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(?:\.[a-zA-Z0-9](?:[a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?)*$/;
$("#first-name-info").html("").hide();
$("#email-info").html("").hide();
if (firstName.trim() == "") {
$("#first-name-info").html("required.").css("color", "#ee0000").show();
$("#first-name").addClass("error-field");
valid = false;
}
if (email == "") {
$("#email-info").html("required").css("color", "#ee0000").show();
$("#email").addClass("error-field");
valid = false;
} else if (email.trim() == "") { $("#email-info").html("Invalid email address.").css("color", "#ee0000") .show();
$("#email").addClass("error-field");
valid = false;>br> } else if (!emailRegex.test(email))
{ $("#email-info").html("Invalid email address.").css("color", "#ee0000")
.show();
$("#email").addClass("error-field");
valid = false;
}
if (valid == false) {
$('.error-field').first().focus();
valid = false;
}
return valid;
}
function Checkout() {
var valid;
valid = validateUser();
if (valid) {
jQuery.ajax({
url : "ajax-endpoint/create-order-endpoint.php", data : 'userName=' + $("#first-name").val() + '&userEmail=' + $("#email").val() + '&productQuantity=' + $("#productQuantity").val(), type : "POST", beforeSend : function() { $("#loader").show(); $("#checkout-btn").hide();
},
success : function(data) { $("#mail-status").html(data);
$('#checkout-form').find('input:text').val('');
$("#loader").hide();
$("#customer-detail").hide();
$("#checkout-btn").show();
}, error : function() {
} }); } } function buynow() { $("#customer-detail").show(); }
checkout via email
This is the first step to set the application configuration to run this example.It sets the product detail in an array which is displayed on the home page dynamically.
It has a constant DISPLAY_QUANTITY_INPUT which is a flag that contains boolean true/false. If it is true, the product tile will allow users to enter the quantity.
config/Config.php
<?php namespace evlearner; /** * This class contains the configuration options */This file is the PHP endpoint process of the AJAX request. It receives the customer and the order details and put an order entry into the database.
class Config
{
const ADMIN_EMAIL = 'admin@gmail.com';
const CURRENCY_CODE = 'USD';
const DISPLAY_QUANTITY_INPUT = true;
public static function getProduct()
{
$products = array(
"productId" => 1,
"name" => "FinePix Pro2 3D Camera",
"price" => 1500.00,
"imageUrl" => 'images/camera.jpg'
);
return $products;
}
}
It instantiates the CheckoutService class to access the order and the order-item database. After placing the order, it invokes the MailService to send the order confirmation via email. In this example, it sends an order confirmation email to the customer. Also, it sends another email to Admin to notify that an order is placed.
ajax-endpoint/create-order-endpoint.php
<?php use evlearner\CheckoutService;This is a complete code of the CheckoutService class in PHP.
use evlearner\Config;
use evlearner\MailService;
require_once __DIR__ .
'/../lib/CheckoutService.php';
require_once __DIR__ .
'/../config/Config.php'; require_once __DIR__ .
'/../lib/MailService.php';
$configModel = new Config();
$configResult = $configModel->getProduct();
$checkoutService = new
CheckoutService();
$mailService = new MailService();
$orderId = $checkoutService->getToken();
$productQuantity = 1;
if (! empty($_POST["productQuantity"])) { $productQuantity = $_POST["productQuantity"];
} $checkoutService->insertOrder($orderId, $_POST["userName"], $_POST["userEmail"], Config::CURRENCY_CODE, $configResult["price"]);
$checkoutService->insertOrderItems($orderId, $configResult["name"], $productQuantity, $configResult["price"]);
$orderResult = $checkoutService->getOrder($orderId);
$orderItemResult = $checkoutService->getOrderItems($orderId);
$recipientArr = array( 'email' => $orderResult[0]["email"] );
$mailService->sendUserEmail($orderResult, $recipientArr);
$mailService->sendAdminEmail($orderItemResult, $orderResult);
?>
It prepares the queries to insert and read the order and order-item entries.
Also, it includes some utility functions to generate a hash token for using it as an order reference id.
lib/CheckoutService.php
<?phpThe MailService class defines to functions separately to send the customer and Admin emails on the placed orders. It uses PHP mail() function for sending emails. You can integrate PHPMailer or any other library instead. If you want a code to send email using PHPMailer via SMTP, the linked tutorial will have the example.
namespace evlearner;
require_once __DIR__ .
'/../config/Config.php';
class CheckoutService
{
private $dbConn;
require_once __DIR__ .
'/../config/DataSource.php';
$this->dbConn = new DataSource();
}
function insertOrder($orderId,
$name, $email, $currency, $amount)
{
$query = "INSERT INTO tbl_order (order_id, name, email, currency, amount) VALUES (?, ?, ?, ?, ?)";
$paramType = "ssssd";
$paramValue = array(
$orderId,
$name,
$email,
$currency,
$amount
);
$insertId = $this->dbConn->insert($query, $paramType, $paramValue);
return $insertId;
}
function insertOrderItems($orderId, $name, $quantity, $item_price)
{
$query = "INSERT INTO
tbl_order_items (order_id, name, quantity, item_price) VALUES (?, ?, ?, ?)";
$paramType = "ssid";
$paramValue = array(
$orderId,
$name,
$quantity,
$item_price
);
$insertId = $this->dbConn->insert($query, $paramType,
$paramValue);
return $insertId;
}
function getOrder($orderId)
{
$query = "SELECT * FROM
tbl_order WHERE order_id='" . $orderId . "'";
$orderResult = $this->dbConn->select($query);
return $orderResult;
}
function getOrderItems($orderId)
{
$query = "SELECT * FROM tbl_order_items WHERE order_id='" . $orderId . "'";
$orderItemResult = $this->dbConn->select($query);
return $orderItemResult;
}
function getToken()
{
$token = "";
$codeAlphabet =
"ABCDEFGHIJKLMNOPQRSTUVWXYZ";
$codeAlphabet .=
"abcdefghijklmnopqrstuvwxyz";
$codeAlphabet .= "0123456789";
$max = strlen($codeAlphabet) - 1;
for ($i = 0; $i < 10; $i ++)
{
$token .= $codeAlphabet[mt_rand(0, $max)]; }
return $token;
}
}
?>
lib/MailService.php
The MailService class defines to functions separately to send the customer and Admin emails on the placed orders.
It uses PHP mail() function for sending emails. You can integrate PHPMailer or any other library instead. If you want a code to send email using PHPMailer via SMTP, the linked tutorial will have the example.
<?php
namespace evlearner;
class MailService
{
function
sendUserEmail($orderResult, $recipientArr)
{
$name = $orderResult[0]["name"];
$email = $orderResult[0]["email"];
$orderId = $orderResult[0]["order_id"];
$subject = "Order Confirmation";
$content = "Hi, you have placed the order successfully, the order reference id is $orderId. We will contact you shortly. Thank you. Regards, Shop Name team.";
$toEmail = implode(',', $recipientArr);
$mailHeaders = "From: " . $name . "<" . $email . ">\r\n";
$response = mail($toEmail, $subject, $content, $mailHeaders);
if ($response) {
echo "<div class='success'><h1>Thank you for shopping with us.</h1>
Your order has been placed and the order reference id is " . $orderId . ".
We will contact you shortly.</div>";
} else
{
echo "<div class='Error'>Problem in Sending Mail.</div>";
}
return $response;
}
function
sendAdminEmail($orderItemResult, $orderResult)
{
$name = $orderItemResult[0]["name"];
$email = $orderResult[0]["email"];
$currency = $orderResult[0]["currency"];
$orderId = $orderItemResult[0]["order_id"];
$price = $orderItemResult[0]["item_price"];
$subject = "New order placed";
$content = "New order is placed and the order reference id is $orderId.\n\nProduct Name: $name.\nPrice: $currency $price";
$toEmail = Config::ADMIN_EMAIL;
$mailHeaders = "From: " . $name . "<" . $email . ">\r\n";
$response = mail($toEmail, $subject, $content, $mailHeaders);
return $response;
}
}
Database script
--
-- Database: `single-product-ecommerce`
--
-- --------------------------------------------------------
--
-- Table structure for table `tbl_order`
--
CREATE TABLE `tbl_order` (
`id` int(11) NOT NULL,
`order_id` varchar(255) NOT NULL,
`name` varchar(255) NOT NULL,
`email` varchar(255) NOT NULL,
`currency` varchar(255) NOT NULL,
`amount` decimal(10,2) NOT NULL,
`create_at` timestamp NOT NULL
DEFAULT current_timestamp() ON
UPDATE current_timestamp()
) ENGINE=InnoDB DEFAULT
CHARSET=utf8mb4;
-- --------------------------------------------------------
--
-- Table structure for table `tbl_order_items`
--
CREATE TABLE `tbl_order_items` (
`id` int(11) NOT NULL,
`order_id` varchar(255) NOT NULL,
`name` varchar(255) NOT NULL,
`quantity` int(11) NOT NULL,
`item_price` decimal(10,2) NOT NULL,
`create_at` timestamp NOT NULL
DEFAULT current_timestamp() ON UPDATE current_timestamp()
) ENGINE=InnoDB DEFAULT
CHARSET=utf8mb4;
--
-- Indexes for dumped tables
--
--
-- Indexes for table `tbl_order`
--
ALTER TABLE `tbl_order`
ADD PRIMARY KEY (`id`);
--
-- Indexes for table `tbl_order_items`
--
ALTER TABLE `tbl_order_items`
ADD PRIMARY KEY (`id`);
--
-- AUTO_INCREMENT for dumped tables
--
--
-- AUTO_INCREMENT for table `tbl_order`
--
ALTER TABLE `tbl_order`
MODIFY `id` int(11) NOT NULL
AUTO_INCREMENT;
--
-- AUTO_INCREMENT for table `tbl_order_items`
--
ALTER TABLE `tbl_order_items`
MODIFY `id` int(11) NOT NULL
AUTO_INCREMENT;
Single Product eCommerce Website results
The following output screenshots above displays the product tile look and feel. Also, it shows the checkout form collecting customer details for the email checkout.By lets see it again !
Comments
Post a Comment